Section Vocab:
- Arithmetic Operators: Standard addition, subtraction, and multiplication operators.
- Comparison Operations: Compares values variables and returns true or false.
- Logical Operators: Used to combine multiple true and false values to return a single true or false value.
Associated Learning Outcome:
At the end of this module students will be able to:
- Identify arithmetic/comparison/logical operators in relation to variables.
Arithmetic Operations
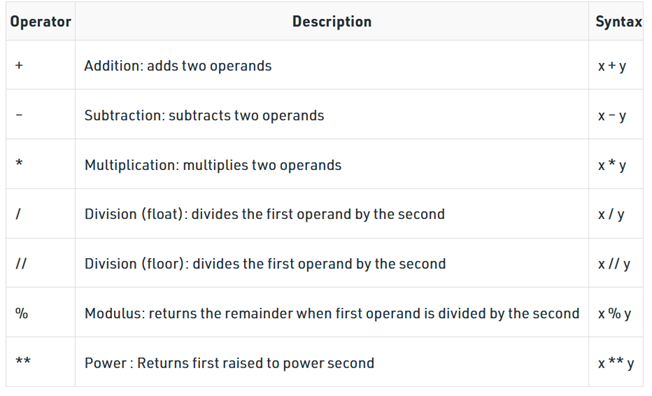
Arithmetic Operators are quite useful in python programming. They are used to carry out mathematical operations such as addition, subtraction, multiplication, and division.
Order of Arithmetic Operations:
The order of operations follows much like BEDMAS in mathematics. The order of operations is listed below with 1 being the fist operations completed and 4 being the last operations completed.
- Brackets ():
- Exponents **:
- Multiplication *, Division (Float) /, Division (Floor) //, Modulus %
- Addition +, Subtraction β
Another note about arithmetic operations is that output type can depend on the two input data types. Some operations work how we expect, like adding two integers outputs an integer; however, other operations may work slightly different than expected, such as dividing integers, “4 / 2” would output the float 2.0.
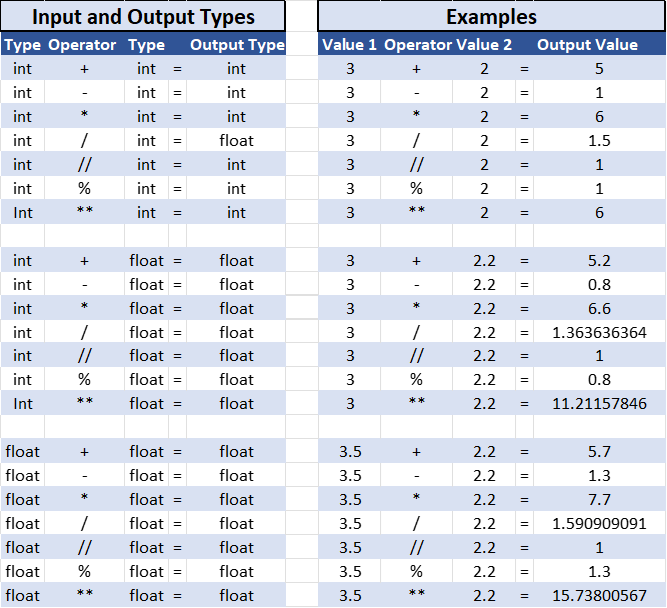
Comparison Operations
Comparison Operations are used to compare values and variables. When two values or variables are compared the result will be a Boolean (True or False).
Letβs take a look at some comparison operators.
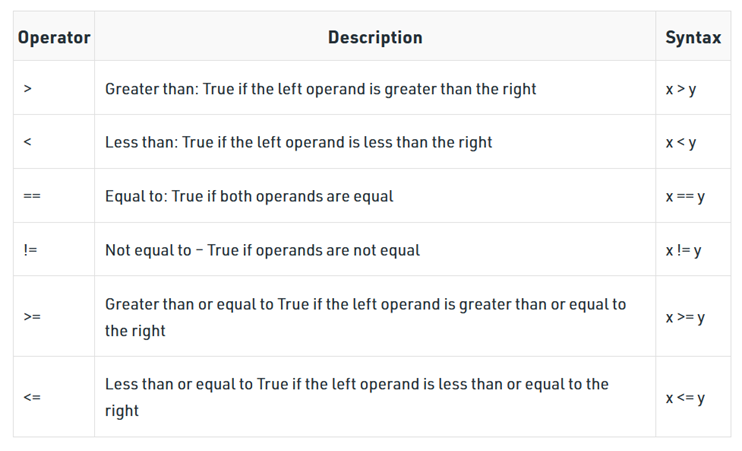
Comparison operations work much like asking a true or false question.
For example:
The statement β4 < 5β is like asking βIs 4 less than 5?β. The answer or output would be True. On the other hand, the statement β5 < 4β is like asking βIs 5 less than 4?β. The answer or output would be False.
The βequal toβ or == is another useful comparison operation. It is used to determine if two values are the same. The statement β4 == 5β is like asking βIs 4 equal to 5β and the output would be False. The statement β4 == 4β is like asking βIs 4 equal to 4β, the answer would be yes, and the output would be True.
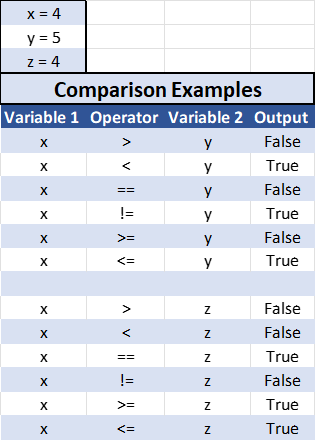
Logical Operations
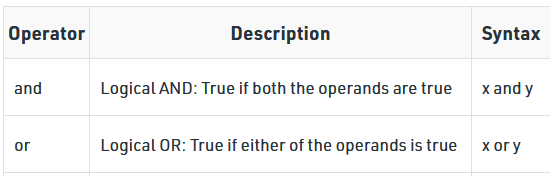
Logical AND and Logical OR are two logical operators that allow us to combine multiple True/False statements to produce a single Boolean output. A logical operator is used between two comparison statements to provide a single Boolean output (True / False).
Building on our example above we can use a logical operator between the two statements,
β4 < 5 and 4 == 5β. This statement would be equivalent to asking, βIs 4 less than 5 and is 4 equal to 5?β. It is true that 4 is less than 5 but it is false that 4 is equal to 5. Since we used the βandβ operator, both elements of the statement must be True for the statement to be True. Therefore, the output for the statement β4 < 5 and 4 == 5β would be False. In contrast, if we used the Logical Or, β4 < 5 or 4 == 5β, the statement would be like asking βIs 4 less than 5 or is 4 equal to 5?β In this case, we used the βorβ operator and if one of the statements is True than the entire statement becomes True. Therefore, the statement β4 < 5 or 4 == 5β is True.
Combining Operations
Combining multiple operators can be an effective method to create complex logic statements. Before we go into an example it is important to note that there is an order in which the operators are evaluated.
Order of operator evaluation:
- First, the arithmetic operators.
- Next, the comparison operators.
- Finally, the logical operators.
Now letβs take a look at the statement β5 + 7 <= 3 * 5 and 3**2 == 18 / 2β.
In order to correctly evaluate the output of this statement we must use our rules for the order of operator evaluation. The first operations evaluated are the arithmetic operations, in this case that would be the β+, *, **, /β. So, β5 + 7β equals 12, β3 * 5β equals 15, β3 ** 2β equals 9.0, and β18 / 2β equals 9.0. After the arithmetic operations are completed, the statement would look like β12 <= 15 and 9.0 == 9.0β.
Next, the comparison operations are assessed, that would be the β<=, ==β. β12 <= 5β is True and β9.0 == 9.0β is True. After the comparison operations are completed, the statement would look like βTrue and Trueβ.
Finally, the logical operator βandβ is considered, βTrue and Trueβ is True.
Therefore, when the statement β5 + 7 <= 3 * 5 and 3**2 == 18 / 2β is evaluated, the output is βTrueβ.
Interactive Activities:
Reading:
Viewing:
References
Analyst, A. T. (2022, Dec). Comparison, Logical, and Membership Operators in Python | Python for Beginners . Retrieved from YouTube: https://www.youtube.com/watch?v=lPVke-p4S7s
ankthon. (2020, Aug 29). Relational Operators in Python. Retrieved from Geeks for Geeks: https://www.geeksforgeeks.org/relational-operators-in-python/
Qingkai Kong, T. S. (2020). Logical Expressions and Operators. Retrieved from Python Mumerical Methods: https://pythonnumericalmethods.berkeley.edu/notebooks/chapter01.05-Logial-Expressions-and-Operators.html