Conditionals:
Section Vocab:
- Conditional Statements: Programming language commands for handling conditions.
- If-statement, If-Else, Elif: Types of conditional statements.
- Operand: An element in a programming language
- NOT: A logical operator that negates a single operand.
- Flow Control: The order in which individual statements are executed
Associated Learning Outcome:
At the end of this module students will be able to:
- Identify the effects of operators within conditional statements
Conditional statements in programming are used to make decisions about what you want your program to do. For simplicity, we will be looking at boolean operators (True/False) combined with conditional statements. In Python, remember to pay attention to indentation, especially when using conditional statements. Weāre going to look at two conditional statements: āifā, and āif-elseā. The general syntax for the if-statement looks like the following:
If:
if <condition>:
<statement>
ā¦.
Note that the if-statement may contain an expression or a condition. The flow control for the if-statement works by first checking the condition inside the if-statement. If it is true, the program will continue inside the if-statement. It will then execute the statement on line 2 above. If the condition turns out to be false, the entire if-statement will be skipped and the program will continue to line 3 above and execute the rest of the program. If we wanted to check that the condition is false, we can add an exclamation mark in front of the condition like so:
if <!condition>:
<statement>
ā¦.
The exclamation mark is a relational operator which means āNOTā. For the example above, we can describe it as āIf NOT this condition, then continue inside the if-statementā or āIf this condition is false, continue inside the if-statementā.
Now letās move on to the if-else statement. The flow control is similar to the if-statement, except with one additional piece of the flow control. The flow control here can be described as āIf this condition is true, continue inside the first block of code (line 2), otherwise, do something else (line 4)ā.
If-else:
if <condition>:
# block of code if condition is True
else:
# block of code if condition is False
Again, we can check if the first condition is false just like we did with the if-statement above by adding an exclamation mark:
if <!condition>:
# block of code if condition is True
else:
# block of code if condition is False
Here is a control-flow diagram to illustrate what happens if the if condition turns out to be true or false:
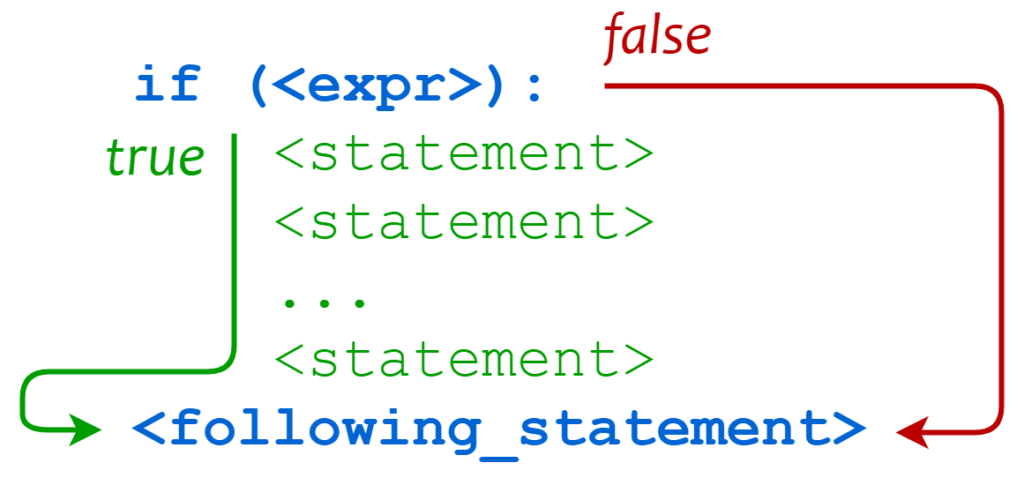
The above image reads the expression, <expr> inside the brackets, and if the <expr> turns out to be true, it will continue executing the green statements inside the if-statement before continuing to execute the rest of the program. If <expr> happens to be false, it will skip the green <statement>’s inside the if-statement to the statements outside of the if-statement and reaches the line <following_statements> to execute the remainder of the program.
Now that we have an understanding of the control flow of conditional statements and how to implement some basic operators, letās combine these two. Letās say we have a variable x set to 1, and we want to check if x is bigger than or equal to 1, and then print āHello Worldā if the condition is true – we can do this by using the if-statement:
x = 1
if (x >= 1):
print(āHello Worldā)
ā¦.
The output will be: Hello World
Since 0 is NOT bigger than or equal to 1, the control flow will continue to line 5 and the output will be: Hello
Again, we can also check that the first condition is false with an exclamation mark:
X = 0
if (x >= 1):
print(āHello World)
else:
print(āHelloā)
Now letās say we want an additional statement in case our condition turns out to be false. If our number is not bigger than or equal to 1, we want to just print āHelloā. We can do this with the help of an if-else statement:
X = 0
if !(x >= 1):
print(āHello World)
else:
print(āHelloā)
Our output will be the same as before.
Elif is another statement that is used in conjunction with the if-statement. Elif is short for āelse ifā. Elif is used to check multiple statements if the first condition is not true.
Here is a flow control chart/syntax for elif:
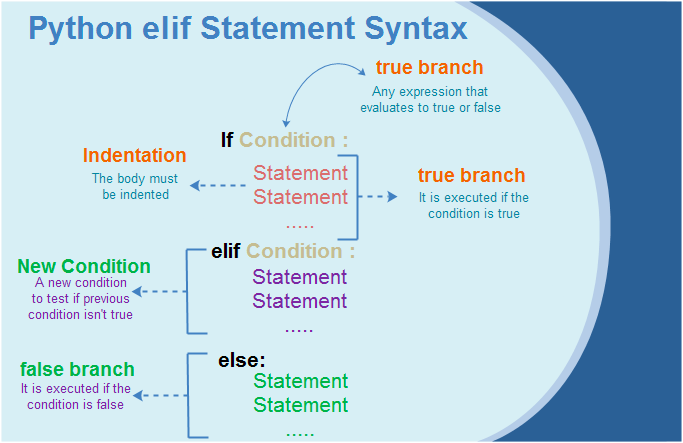
The flow control reads the condition next to ‘If’; if the condition is true, it will continue executing the red statements inside the if-statement, and if it is false, it will continue to the ‘elif Condition’. If the condition next to elif happens to be true, it will continue to execute the purple statements inside the ‘elif Condition’ block, and if the condition happens to be false, it will continue to the ‘else:’ line. If the condition next to the ‘If’ and ‘elif’ statement happens to be false, it will execute the green statements inside the ‘else’ block.
Examine conditionals in context within the calculator program:
Can you point out where the conditional statements are?
Can you alter the conditional statements to make the calculator output something different?
Optional
Copy the code from here!
Press shift+enter or the little play button in the top left corner to run (execute) the program.
Paste it above (in the little grey box next to [ ]:
)!
When you are ready, watch the YouTube video and answer the assessment:
https://pythonnumericalmethods.berkeley.edu/notebooks/chapter04.01-If-Else-Statements.html (optional reading)
https://www.educative.io/answers/what-are-conditional-statements-in-programming (optional reading)